How to Prepare for Coding Interviews: Tips & Strategies
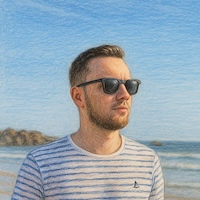
Max
The Hidden Psychology of Coding Interviews
Landing a remote tech job often depends on how you perform in the coding interview. But it’s not just about technical prowess. It’s about the intersection of psychology, clear communication, and your problem-solving abilities. Grasping this hidden dynamic can significantly improve your interview performance. It’s about going beyond just coding and recognizing what interviewers truly want to see.
What Interviewers Are Really Looking For
Of course, technical skills are essential. However, interviewers also assess your problem-solving approach, your communication style, and how you perform under pressure. For example, explaining your thought process is just as important as writing flawless code. This shows you can collaborate effectively and explain technical concepts to non-technical colleagues. How you handle unexpected challenges also reveals your resilience and adaptability – key traits for remote work.
Preparing for these interviews can be tough, especially for those just starting out. In fact, over 60% of software engineers consider coding interviews the most stressful part of the job search. Newcomers to coding are often advised to spend 3 to 6 months preparing. You can find more detailed statistics here: Learn more about coding interview prep. This emphasizes the importance of a structured and personalized preparation strategy.
Different Interview Formats and How to Prepare
Coding interviews come in various formats, each with unique psychological hurdles.
Whiteboard Coding: This classic format tests your ability to think on your feet and explain your logic clearly. Practicing on a physical or digital whiteboard can build confidence and fluency.
Take-Home Challenges: These evaluate your ability to write clean, efficient code in a less pressured environment. Prioritize code readability, documentation, and testing.
Pair Programming: This format assesses your collaboration and communication skills in a real-world scenario. Practice with others to improve how you articulate your thoughts and incorporate feedback.
Successfully navigating these formats means understanding the subtle factors that influence interviewer decisions. It’s not enough to just know the format; understanding the nuances of each interview type will greatly increase your confidence and improve your performance. This translates to a more comprehensive and effective preparation strategy, maximizing your chances of landing that remote role.
Your Personalized Interview Preparation Blueprint
One-size-fits-all interview prep just doesn’t cut it in the coding world. Every candidate brings a unique mix of experience, strengths, and weaknesses to the table. This section offers a personalized framework to help you build a winning interview strategy. We’ll factor in your background, available time, and target companies to create a roadmap that works for you. We’ll also explore realistic timelines, from one-month sprints to six-month deep dives, and highlight key milestones to keep you on track.
Prioritization and Effective Time Allocation
Successful candidates know how to prioritize. Don’t try to conquer everything at once. Instead, focus on the core concepts that pop up most often in interviews. For example, mastering array manipulation and linked lists will generally be more valuable than getting lost in niche algorithms early on.
One common roadblock is feeling stuck. The same principles that apply to overcoming writer’s block also apply to coding. Consistent practice, even in short bursts, is more effective than sporadic cramming.
Block out specific times for learning new concepts.
Schedule dedicated practice sessions for coding problems.
Set aside time for reviewing solutions and identifying areas for improvement.
This structured approach will keep you focused and prevent burnout.
Learning From Success Stories
Learn from those who have already walked the path. Engineers who’ve landed roles at top tech companies often stress the importance of balancing breadth and depth of knowledge. This means having a broad understanding of various concepts while deeply mastering the most relevant ones. For example, a front-end developer might prioritize JavaScript frameworks and UI/UX principles while still maintaining a solid grasp of core algorithms. This targeted approach optimizes your preparation for specific roles and companies. By analyzing your strengths, weaknesses, and available time, you can create a personalized blueprint for interview success. This personalized approach allows you to maximize your efforts and boost your chances of landing your dream role.
Data Structures & Algorithms: The Strategic Approach
Mastering data structures and algorithms (DS&A) is essential for success in coding interviews. It’s not just about memorization; it’s about deeply understanding how these fundamental components function. This section will guide you through a strategic approach that successful candidates use to prepare for this crucial interview element. We’ll explore essential data structures like arrays, linked lists, trees, and graphs, along with key algorithms such as sorting, searching, and dynamic programming.
Essential Data Structures and Their Applications
Choosing the right data structure for a specific problem is paramount. An array, for instance, with its contiguous memory allocation, is excellent for quickly accessing elements by index. However, inserting or deleting elements within an array can be computationally costly.
This is where linked lists shine. While they don’t offer direct index access, they provide efficient insertion and deletion capabilities. The best choice between these two often hinges on the problem’s specific requirements.
This “right tool for the job” principle also applies to more complex structures like trees and graphs. Trees, with their hierarchical structure, are perfect for representing hierarchical data and enabling efficient searching and sorting. Graphs, conversely, represent relationships between entities, finding applications in areas like social networks and mapping systems.
Mastering Algorithms for Problem Solving
Understanding different algorithms and their complexities helps you write efficient, optimized code. Sorting algorithms like quicksort or mergesort have different performance characteristics depending on the data. Selecting the right sorting algorithm can significantly impact your solution’s efficiency.
Searching algorithms such as binary search or depth-first search are optimized for specific data structures and scenarios. Knowing when to use each algorithm showcases your ability to write streamlined, efficient code.
Dynamic programming solves problems by breaking them down into smaller, overlapping subproblems. This method optimizes performance by storing and reusing the results of these subproblems, preventing redundant calculations. Mastering this technique often sets strong candidates apart. You might be interested in: How to master remote talent acquisition.
Strategic Learning and Common Pitfalls
Developing intuition about DS&A is key for tackling unfamiliar problems. This involves understanding the underlying principles, not just memorizing implementations. A reasonable timeframe for mastering these concepts is typically 3-6 months. This allows ample time to learn programming basics, understand fundamental DS&A, and progress to more complex problems. In the final months, mock interviews are crucial, simulating real interview conditions and refining problem-solving skills under pressure. Explore this topic further: Discover more insights about coding interview prep.
One common pitfall is focusing only on implementation without understanding the why behind choosing a specific data structure or algorithm. Interviewers value candidates who can articulate their reasoning and explain the trade-offs. Another mistake is neglecting edge cases. Testing your code thoroughly with various inputs, including boundary conditions and invalid data, demonstrates attention to detail and solid coding practices.
Problem-Solving Frameworks That Impress Interviewers
Acing coding interviews hinges on more than just writing correct code. It’s about showcasing a structured, methodical approach to problem-solving. This is what truly sets exceptional candidates apart. This section explores the frameworks employed by engineers who consistently shine in these high-stakes interviews. We’ll look at how they dissect problems, articulate their thinking, and build solutions that highlight their skills. For developers preparing for interviews, consider exploring these helpful resources: AI Tools for Coding.
Breaking Down Problems Methodically
A systematic approach is essential for effective problem-solving. Begin by fully understanding the problem. Ask clarifying questions to remove any ambiguity and demonstrate your analytical abilities.
For example, if asked to reverse a linked list, inquire about handling empty lists or those with only one element. This shows you consider edge cases, a hallmark of a thorough approach. Restate the problem in your own words to confirm you and the interviewer are on the same page. This reinforces your understanding and highlights your communication skills.
Next, decompose the problem into smaller, more manageable parts. This makes the task less intimidating and allows you to target solutions for each component. This modular approach simplifies coding and debugging.
Communicating Your Thought Process Effectively
Articulating your thoughts clearly is as important as writing functional code. Talk through your reasoning as you break down the problem and explore potential solutions. This gives the interviewer valuable insight into your problem-solving strategy and allows them to offer guidance if needed.
Avoid rambling. Focus on conveying your logic concisely and effectively. This demonstrates your ability to communicate technical concepts with clarity. Discuss the time and space complexity of your proposed solutions. This showcases your understanding of algorithm efficiency and your ability to write optimized code. For example, explain your choice of a specific sorting algorithm based on its time complexity and relevance to the problem’s constraints.
Building Solutions and Handling Challenges
With a plan in place, begin coding, but avoid rushing. Write clean, well-documented code, even under pressure. Use descriptive variable names and comments to clarify your code’s logic. This not only demonstrates good coding practices but also makes it easier for the interviewer to understand your work.
If you encounter an obstacle, don’t panic. Explain your current approach, pinpoint where you’re stuck, and consider alternative strategies. This shows resilience and adaptability, valuable traits in any engineer. Finally, test your code thoroughly. Address edge cases and explain how your solution handles various scenarios. This demonstrates attention to detail and a commitment to delivering robust, functional code.
By mastering these problem-solving frameworks, you can turn coding interviews from stressful hurdles into opportunities. This structured approach allows you to solve problems efficiently and highlights the qualities interviewers seek, significantly boosting your chances of success.
Daily Practice Routines of Successful Candidates
Consistent, deliberate practice is essential for acing coding interviews. This section explores the routines of successful candidates at top tech companies, revealing how they structure their practice, monitor progress, and maximize learning from each problem. It’s not about mindless repetition; it’s about practicing with intention.
Designing Effective Practice Sessions
Effective practice prioritizes quality over quantity. Design sessions that challenge you appropriately, starting with problems slightly beyond your current skill level. This pushes your boundaries without feeling overwhelming.
Focus on understanding: Don’t just memorize solutions. Break down the problem, grasp the core concepts, and articulate the logic behind your solution.
Time yourself: Simulate the pressure of a real interview by setting time limits. This improves your speed and helps you manage stress during the actual interview.
Vary problem types: Avoid getting stuck in a rut. Tackle diverse problems involving different data structures and algorithms. This strengthens your overall problem-solving abilities.
For example, dedicating at least an hour daily to selected practice questions improves familiarity with common interview problems. Consistent practice and exposure to various question types are crucial for a positive interview experience. Explore this topic further. You might also find this helpful: How to master remote work best practices.
Powerful Techniques for Deeper Learning
Certain techniques significantly improve learning and retention. Spaced repetition, reviewing material at increasing intervals, solidifies knowledge in long-term memory.
The Feynman Technique, explaining a concept as if teaching it, reveals knowledge gaps and reinforces understanding. Deliberate practice involves identifying weaknesses and systematically addressing them. This focused approach maximizes improvement, building both speed and accuracy.
This active approach is far more effective than passive review. By actively engaging with the material and striving for deep understanding, you build a solid foundation for tackling interview questions.
Utilizing the Right Platforms and Simulating Interview Conditions
Choosing the right practice platform is essential. Platforms like LeetCode, HackerRank, and Codewars offer extensive libraries of interview-relevant problems.
LeetCode: Known for its vast collection of algorithm problems frequently asked by top tech companies.
HackerRank: Popular for its contests and skill assessments, often used by companies in the hiring process.
Codewars: Offers a gamified approach to learning and practicing coding skills.
Simulate real interview conditions by practicing on a whiteboard or using a collaborative coding platform with a friend. This recreates the interview environment, building your comfort level in explaining your thought process verbally. This preparation sharpens your technical skills while enhancing communication and problem-solving abilities, setting you up for success. These combined practices create a strong base for effectively tackling coding challenges.
Technical Communication That Sets You Apart
Your code is only half the battle in a coding interview. What truly distinguishes you is your ability to articulate your code, your problem-solving process, and your technical choices. This section explores how top performers effectively convey intricate technical concepts, even under pressure. This communication skill can be the deciding factor between candidates with similar qualifications.
Verbalizing Your Thought Process
Consider your interviewer a collaborator, not just an assessor. Guide them through your solution step by step, verbalizing your thought process along the way. This demonstrates your problem-solving methodology and instills confidence in your capabilities. For example, when choosing between a breadth-first search and a depth-first search, explain your rationale based on the specific problem.
Explain your technical decisions persuasively. Justify your choice of a particular data structure or algorithm clearly and concisely. This highlights your grasp of the inherent trade-offs and your ability to make informed decisions.
Responding to Feedback and Asking Insightful Questions
Responding constructively to feedback is crucial. If the interviewer suggests an alternative approach, remain receptive. Listen attentively, ask clarifying questions if necessary, and show your adaptability. This flexibility is highly valued in remote work settings.
Asking insightful questions demonstrates your engineering mindset. Don’t hesitate to inquire about the company’s technical hurdles, coding standards, or team dynamics. This shows genuine interest and initiative. You might find this helpful: How to master virtual interviews.
Finding the Balance Between Technical Precision and Accessibility
While technical accuracy is paramount, avoid overly complicated explanations. Prioritize clarity and accessibility. Use analogies and examples to illustrate complex concepts in an easily digestible manner. This shows your ability to communicate with both technical and non-technical audiences.
Imagine explaining recursion using the analogy of Russian nesting dolls, each containing a smaller version of itself. This simplifies a complex concept. Similarly, real-world examples of how a specific algorithm functions can make your explanation more engaging and memorable.
Communication: The Deciding Factor
Technical communication isn’t simply about speaking clearly; it’s about presenting your ideas persuasively, building confidence, and showcasing your problem-solving skills. This often separates successful candidates from the rest. Mastering these communication techniques can transform your coding interviews from mere technical tests into compelling displays of your skills and potential, unlocking opportunities in the remote job market.
Specialized Preparation for Your Target Role
Preparing for coding interviews isn’t one-size-fits-all. Different roles require different strategies, so focus on the specific skills and technologies relevant to your target domain. This section offers targeted guidance for front-end development, back-end engineering, full-stack positions, mobile application development, data science, and machine learning roles.
Front-End, Back-End, and Full-Stack Development
For front-end roles, mastering JavaScript frameworks is essential. Think React, Angular, or Vue.js. A strong grasp of HTML, CSS, and responsive design principles is also crucial. Interviewers often assess your ability to build user interfaces and implement interactive features.
Back-end engineers should focus on server-side languages like Python, Java, or Node.js. Database management, API design, and security best practices are also key areas to study. Expect questions about system architecture and scalability.
Full-stack developers need a solid foundation in both front-end and back-end technologies. Demonstrating proficiency in a particular tech stack, such as MERN (MongoDB, Express.js, React, Node.js) or LAMP (Linux, Apache, MySQL, PHP), can significantly improve your chances.
Mobile Application Development
Mobile developers typically specialize in either iOS or Android development. For iOS, this involves mastering Swift or Objective-C and understanding the iOS SDK. Android developers focus on Java or Kotlin and the Android SDK. Interviewers often assess your experience with mobile-specific concepts like UI design patterns, performance optimization, and platform-specific APIs.
Data Science and Machine Learning
Data science and machine learning roles require strong programming skills, often in Python or R. A deep understanding of statistical modeling, data analysis techniques, and machine learning algorithms is also essential. Preparation isn’t limited to coding; understanding the broader context of the role is important. For data science and statistics roles, Python is often crucial for data analysis and processing. Want more insights about statistics interview questions? Explore this topic further.
Researching Company-Specific Interview Patterns
Understanding a company’s interview style can give you a significant advantage. Reach out to your network, connect with people who have interviewed at your target companies, and gather insights about their experiences. This inside information can offer valuable clues about the types of questions asked, the preferred coding languages, and the overall interview format.
Decoding Job Descriptions and Aligning with Company Culture
Job descriptions are packed with information. Carefully analyze the required skills and experience to identify hidden requirements. This helps you tailor your preparation to the specific needs of the role.
Research the company’s engineering culture and values. Understanding their priorities helps you position your experience to highlight transferable skills. Balancing general coding preparation with specific domain expertise is crucial. Recruiters and hiring managers emphasize showcasing both your fundamental coding skills and your deep understanding of your chosen field.
Start your remote job search today with Remote First Jobs! Explore thousands of remote opportunities and find your perfect fit in the world of remote work.